Embedded Sending
When you integrate with PandaDoc API, you can embed a document right on the your website or in an app. This way, your users can sign or edit a document without leaving your platform.
Embedded Sending use case offers a middle ground between a full document editor and signing - interactive field placement. This is especially useful in these cases:
- You have a PDF generated elsewhere.
- You want to prevent customers from modifying document content while simultaneously allowing to place and adjust fields and recipients.
Customer view
For a customer on your website, the flow looks as follows:
- Upload a PDF using a button.
- Drag and drop document fields on the PDF content. Assign them to recipients.
- Send the resulting PandaDoc document out for signing: send via email, create a signing link, create a session id for Embedded Signing, or sign in person.
- Optionally, save the field configuration to use the exact same placement on another document upload.
Implementation
We have created a simplified version of the document editor to embed into your web application. It is distributed as a JavaScript file that loads an iframe with the editor. To render a document for field placement, you only need to call a JS function with documentId
, token
, workspace_id
, and organization_id
.
To obtain workspace_id
and organization_id
, go to Dev Tools in your browser on app.pandadoc.com page, and execute the next code in console:
global_config.organization
(for organization_id)
global_config.workspace
(for workspace_id)
The token
is a Bearer token you can either get the same way from global_config.token
(for testing purposes only - this token is your personal token), or by using an API endpoint (POST public/v1/members/{member_id}/token
) (it's not available by default, please reach out to your assigned CSM or AM to get it enabled for you).
We are going to simplify this in future and reduce number of parameters.
Embedded Sending demo
The video below shows a demo application we've built to showcase the embedded sending flow:
-
The bottom half of the screen is our embedded editor, that is, the JavaScript snippet mentioned earlier. You pass a
DocumentId
to it dynamically after the document is created. You will then be able to place fields on top of the document and assign a recipient to each of the fields. -
The top part of the screen is an example application that is just one option of how you can plug the embedded editor into your workflow, leveraging the existing PandaDoc API. Here's what our customers typically implement:
- "Upload a PDF" button
- Document lister to display a list of documents
- Defining recipients before creating a document to use recipient data in the document created from a PDF
- Optionally, get fields configuration from the document and apply it to another document. Learn more about this functionality: Replicate fields placement .
See our suggestions for the optimal use case flow below.
Reference architecture
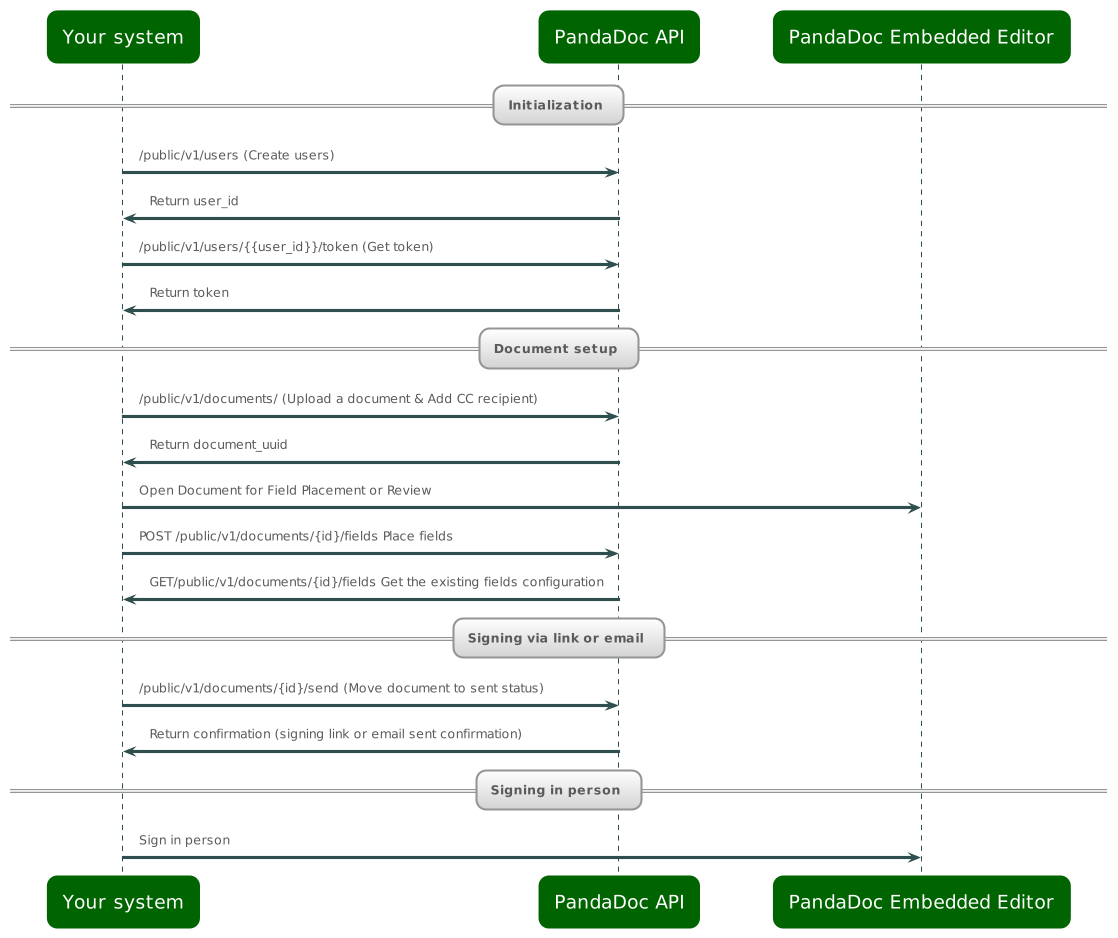
Requirements
The end user's browser must support the postMessage
feature. Most modern browsers support postMessage
.
Getting started
<!DOCTYPE html>
<html>
<body>
<!-- 1. The PandaDoc editor <iframe> will replace this <div> tag. -->
<div id="editor"></div>
<script>
// 2. Creates a new script tag and load the IFrame Editor API code asynchronously.
const tag = document.createElement("script");
tag.src =
"https://static.prod.pandadoc-static.com/prod/es/iframe-editor-api.js";
const firstScriptTag = document.getElementsByTagName("script")[0];
firstScriptTag.parentNode.insertBefore(tag, firstScriptTag);
// 3. This function creates a PandaDoc editor <iframe> after the API initialized.
let editor;
function onPandaDocEditorIframeAPIReady() {
editor = new PD.Editor("editor", {
documentId: "", // Document id
token: "", // Bearer token
workspaceId: "", // Workspace id
organizationId: "", // Organization id,
fieldPlacementOnly: false, //passing true will disable blocks and leave only fillable fields
});
}
</script>
</body>
</html>
Description of the example:
- Placeholder for PandaDoc editor: We start by creating a
<div>
tag with the editorid
. Thisdiv
element serves as the placeholder to insert the PandaDoc editor iframe. IFrame Editor API replaces the<div>
tag with the<iframe>
tag. - Load the IFrame Editor API: We create a new script tag and load the IFrame Editor API code asynchronously. This script tag is inserted before the first script tag in the document.
- Create the PandaDoc editor: After the API is initialized, we create a new PandaDoc editor iframe. This is done inside the
onPandaDocEditorIframeAPIReady
function. The editor is created with the id of the placeholder div and a configuration object. The configuration object includes the document id, bearer token, workspace id, and organization id.
Replicate fields placement
Suppose you already have document templatization in a different tool, and all documents that come to you in a PDF format have slightly different wording but require fields to be placed on exactly the same coordinates. You don't want to place fields manually in the editor every time. What you want to do is to place fields once and then save this layout as a configuration of fields corresponding to a template. You will effectively have two layers:
- A layer of fields that you place on top.
If you save a fields layout separately, you can place it on top of a different PDF as many times as you want. Our clients usually provide a functionality in their application that saves a fields layer as a template that their clients can then reuse. You might even provide a functionality to store several fields templates corresponding to different types of commonly used documents.
Here are the endpoints you can use to develop this functionality:
- List Document Fields to get fields configuration.
- Create Document Fields to apply fields configuration to a document.
Updated 5 days ago