Embedded Sending
When you integrate with the PandaDoc API, you can embed a document directly on your website or in your app. This allows your users to sign or edit a document without leaving your platform.
The Embedded Sending use case offers a middle ground between a full document editor and a signing experience by providing interactive field placement. This is especially useful in the following scenarios:
- You have a PDF generated elsewhere.
- You want to prevent customers from modifying document content while still allowing them to place and adjust fields and edit recipients data.
Useful links
Customer view
For a customer on your website, the flow is as follows:
- Upload a PDF using a button.
- Drag and drop document fields onto the PDF content. Assign these fields to recipients.
- Send the resulting PandaDoc document out for signing using one of these methods:
- Send via email
- Create a signing link
- Create a session ID for Embedded Signing
- Enable in-person signing
- Optionally, save the field configuration to use the exact same placement on future document uploads.
Embedded Sending demo
The video below shows a demo application we've built to showcase the embedded sending flow:
-
The bottom half of the screen is our embedded editor. You can find detailed instruction under bellow Installation section. After that you will be able to place fields on top of the document and assign a recipient to each of the fields.
-
The top part of the screen is an example application that is just one option of how you can plug the embedded editor into your workflow, leveraging the existing PandaDoc API. Here's what our customers typically implement:
- "Upload a PDF" button
- Document lister to display a list of documents
- Defining recipients before creating a document to use recipient data in the document created from a PDF
- Optionally, get fields configuration from the document and apply it to another document. Learn more about this functionality: Replicate fields placement .
See our suggestions for the optimal use case flow below.
Reference architecture
Main flow
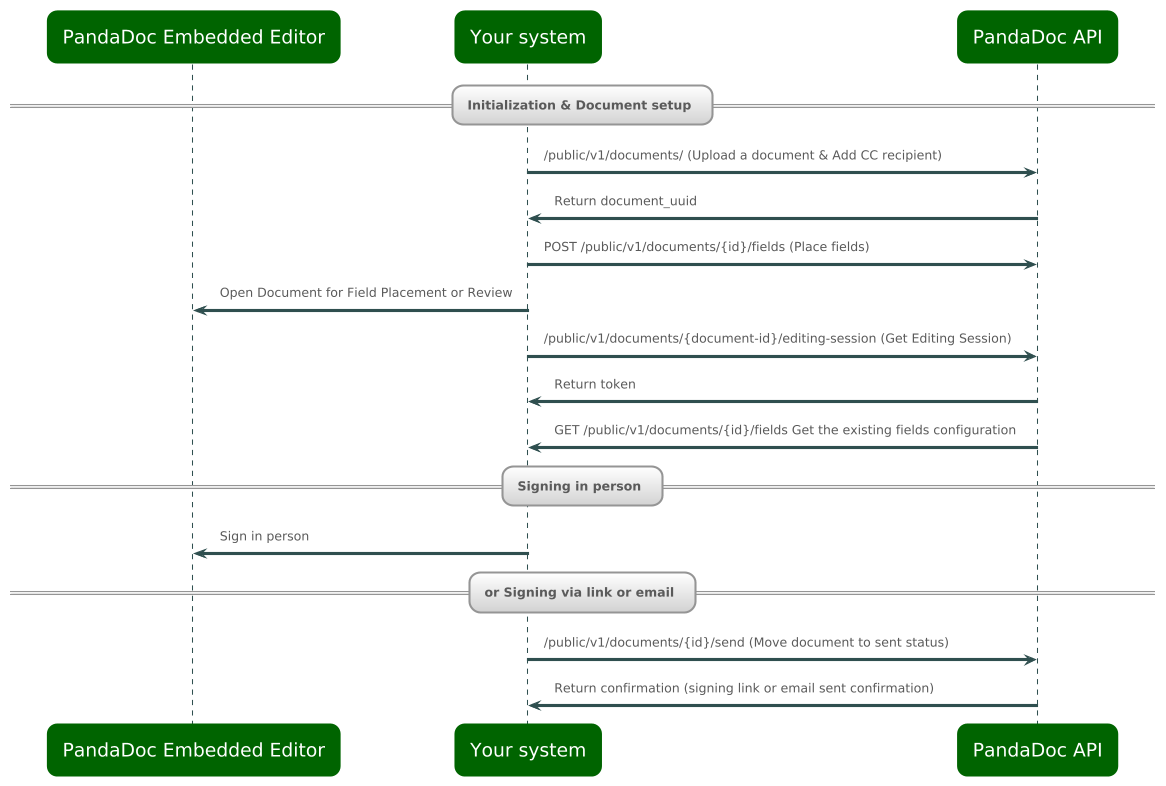
Requirements
The end user's browser must support the postMessage
feature. Most of the modern browsers do support postMessage
.
Implementation
You can embed the PandaDoc Editor in your application by following the steps below.
Step 1: Obtain the editing session key (E-Token)
Use the following API endpoint to get the E-Token
Create Template Editing Session
Create Document Editing Session
Note: These endpoint are not available by default. Please reach out to your assigned CSM or AM to get it enabled for you.
Step 2: Initialize the Editor
PandaDoc Embedded Editor is available as an npm package. Read more
First, install the package:
yarn add pandadoc-editor
After that, you can use it in your project:
import { Editor } from "pandadoc-editor";
Then, you need to initialize the editor.
const editor = new Editor(
"editor-container",
{
fieldPlacementOnly: false, // Enable Blocks
// You can initiate editor loading even without token
token: "", // Edit session E-Token
}
);
After initializing the editor, you must call open
method for the editor to be displayed:
editor.open({
token: "Edit session token"
});
Control Visible Fields
You can control the visibility of fields in the Editor by the 'fields' parameter.
Fields can be set as part of the Editor configuration if you don't need to configure them for each open call.
editor.open({
fieldsPlacementOnly: false,
fields: {
"signature": { visible: false },
"stamp": { visible: false },
"payment_details": { visible: false },
},
});
The 'fields' parameter is an object where the keys are the types of fields you want to control, and the values are objects with a 'visible' property that determines whether the field is visible.
Default field configuration
If you don't provide a field configuration, the default configuration or the configuration that was passed during Editor initialization will be used.
All fields are visible by default.
const defaultFieldConfig = {
"checkbox": { visible: true },
"dropdown": { visible: true },
"date": { visible: true },
"signature": { visible: true },
"stamp": { visible: true },
"initials": { visible: true },
"payment_details": { visible: true },
"collect_file": { visible: true },
"radio_buttons": { visible: true },
};
Control Visible Blocks
You can control the visibility of blocks in the Editor by the 'blocks' parameter.
Blocks can be set as part of the Editor configuration if you don't need to configure them for each open call.
editor.open({
fieldsPlacementOnly: false,
documentId: "", // Document id
blocks: {
"pricingTable": { visible: true },
"page-break": { visible: true },
"quote": { visible: true },
}
});
The 'blocks' parameter is an object where the keys are the types of blocks you want to control, and the values are objects with a 'visible' property that determines whether the block is visible.
Default block configuration
If you don't provide a block configuration, the default configuration or the configuration that was passed during Editor initialization will be used.
All blocks are hidden by default. Pass fieldsPlacementOnly: false
to show all blocks.
const defaultBlockConfig = {
"text": { visible: false },
"image": { visible: false },
"video": { visible: false },
"table": { visible: false },
"pricingTable": { visible: false },
"page-break": { visible: false },
"tableOfContents": { visible: false },
"placeholder": { visible: false },
"quote": { visible: false },
};
Replicate fields placement
Suppose you already have document templatization in a different tool, and all documents that come to you in a PDF format have slightly different wording but require fields to be placed on exactly the same coordinates. You don't want to place fields manually in the editor every time. What you want to do is to place fields once and then save this layout as a configuration of fields corresponding to a template. You effectively have two layers:
- A layer of fields that you place on top of it
If you save a fields layout separately, you can place it on top of a different PDF as many times as you want. Our customers usually provide a functionality in their application that saves a fields layer as a template that their clients can then reuse. You can also provide a functionality to store several fields templates corresponding to different types of commonly used documents.
Here are the endpoints you can use to develop this functionality:
- List Document Fields to get fields configuration.
- Create Document Fields to apply fields configuration to a document.
Updated about 1 month ago