Embedded Editing
The PandaDoc Embedded Editor allows you to edit PandaDoc Documents & PandaDoc Templates without leaving your application.
Useful Links
Requirements
The end user's browser must support the
Windows API: postMessage
. Check Browsers support.
Document Embedded Editing UML flow diagram
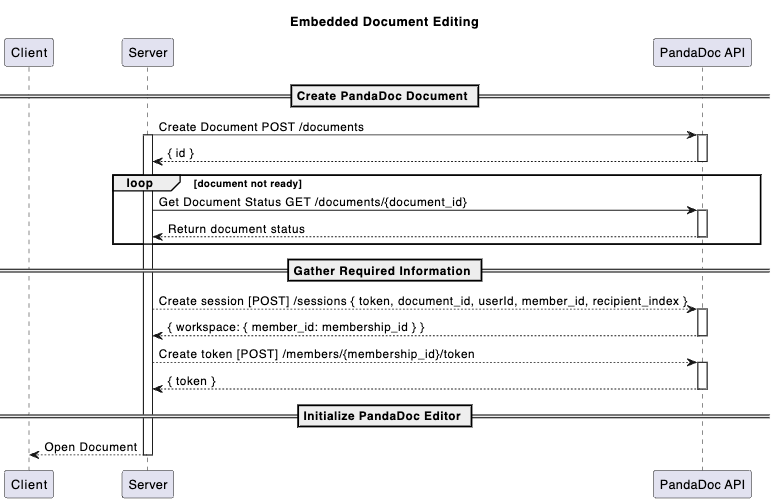
Template Embedded Editing UML flow diagram
Implementation
You can embed the PandaDoc Editor in your application by following the steps below.
Step 1: Gather Required Information
To start the Embedded Editor, you need the following information:
organizationId
workspaceId
documentId
ortemplateId
token
Step 1.1: Obtain workspaceId
and organizationId
workspaceId
and organizationId
If you don't have the workspaceId
or organizationId
, you can obtain them by following the steps below:
- Open https://app.pandadoc.com in your browser.
- Open DevTools in your browser (Press F12).
- Execute the following code in the console to get the
organizationId
:
global_config.organization
- Execute the following code in the console to get the
workspaceId
:
global_config.workspace
Step 1.2: Obtain the token
token
In PandaDoc all modifications in editor are associated with user (using membership in workspace).
You can obtain the member token
in two ways:
Use your token:
- Execute the following code in the console:
javascript global_config.token
- Note: This token is your personal token and should be used for testing purposes only.
Create new token for the Member:
- Use the following API endpoint to get the token Get Member Token
- Note: This endpoint is not available by default. Please reach out to your assigned CSM or AM to get it enabled for you.
Step 2: Initialize the Editor
You can start the Embedded Editor in two ways:
Using npm package
PandaDoc Embedded Editor is available as an npm package. Read more
First, install the package:
npm i pandadoc-editor
After that, you can use it in your project:
import { Editor } from "pandadoc-editor";
Then, you can initialize the editor. Editor will start automatically if you provide documentId or templateId.
const editor = new Editor(
"editor-container",
{
fieldPlacementOnly: false, // Enable Blocks
// Optional parameters:
token: "", // Bearer token
workspaceId: "", // Workspace id
organizationId: "", // Organization id,
documentId: "", // Document id
templateId: "", // Template id
}
);
You can also open a document using openDocument
method:
editor.openDocument({
documentId: "", // Document id
});
Or open a template using openTemplate
method:
editor.openTemplate({
templateId: "", // Template id
});
Using PandaDoc hosted script
Embedding PandaDoc Editor using the script from PandaDoc.
- Start by creating a
<div>
tag with the editorid
. Thisdiv
element serves as the placeholder to insert the PandaDoc editor iframe. - Then create a new script tag and load the IFrame Editor API code asynchronously.
- You can initialize Editor once
onPandaDocEditorIframeAPIReady
function is called.
<!DOCTYPE html>
<html>
<body>
<!-- 1. The PandaDoc editor <iframe> will replace this <div> tag. -->
<div id="editor"></div>
<script>
// 2. Creates a new script tag and load the IFrame Editor API code asynchronously.
const tag = document.createElement("script");
tag.src =
"https://static.prod.pandadoc-static.com/prod/es/iframe-editor-api.js";
const firstScriptTag = document.getElementsByTagName("script")[0];
firstScriptTag.parentNode.insertBefore(tag, firstScriptTag);
let editor;
// 3. This function creates a PandaDoc editor <iframe> after the API initialized.
function onPandaDocEditorIframeAPIReady() {
editor = new PD.Editor("editor", {
fieldPlacementOnly: false, // Enable Blocks
documentId: "", // Document id
token: "", // Bearer token
workspaceId: "", // Workspace id
organizationId: "", // Organization id,
});
}
</script>
</body>
</html>
Control Visible Fields
You can control the visibility of fields in the Editor by the 'fields' parameter.
Fields can be set as part of the Editor configuration if you don't need to configure them for each openDocument/openTemplate call.
editor.openDocument({
documentId: "", // Document id
fieldsPlacementOnly: false,
fields: {
"signature": { visible: false },
"stamp": { visible: false },
"payment_details": { visible: false },
},
});
The 'fields' parameter is an object where the keys are the types of fields you want to control, and the values are objects with a 'visible' property that determines whether the field is visible.
Default field configuration
If you don't provide a field configuration, the default configuration or the configuration that was passed during Editor initialization will be used.All fields are visible by default.
const defaultFieldConfig = {
"checkbox": { visible: true },
"dropdown": { visible: true },
"date": { visible: true },
"signature": { visible: true },
"stamp": { visible: true },
"initials": { visible: true },
"payment_details": { visible: true },
"collect_file": { visible: true },
"radio_buttons": { visible: true },
};
Control Visible Blocks
You can control the visibility of blocks in the Editor by the 'blocks' parameter.
Blocks can be set as part of the Editor configuration if you don't need to configure them for each openDocument/openTemplate call.
editor.openDocument({
fieldsPlacementOnly: false,
documentId: "", // Document id
blocks: {
"pricingTable": { visible: true },
"page-break": { visible: true },
"quote": { visible: true },
}
});
The 'blocks' parameter is an object where the keys are the types of blocks you want to control, and the values are objects with a 'visible' property that determines whether the block is visible.
Default block configuration
If you don't provide a block configuration, the default configuration or the configuration that was passed during Editor initialization will be used.All blocks are hidden by default. Pass fieldsPlacementOnly: false
to show all blocks.
const defaultBlockConfig = {
"text": { visible: false },
"image": { visible: false },
"video": { visible: false },
"table": { visible: false },
"pricingTable": { visible: false },
"page-break": { visible: false },
"tableOfContents": { visible: false },
"placeholder": { visible: false },
"quote": { visible: false },
};
Updated 2 days ago