Embedded Signing
Embed a PandaDoc document and invite customers to sign documents right on your website or in an app, without them having to check email.
Useful links
Here’s how you create a wireframe to embed a document:
Generate a session ID
Your application must perform this sequence of calls:
- Create a document from a template or a PDF:
POST https://api.pandadoc.com/public/v1/documents
. Fetchdocument_id
from the response. - Since the Create Document endpoint is asynchronous, make sure that your document is ready for embedding.
The preferred way to do this is to wait for thedocument_created
webhook.
Alternatively, usedocument_id
to call Document Status until the document status isdocument.draft
:GET https://api.pandadoc.com/public/v1/documents/{document_id}
. - Send your document:
POST https://api.pandadoc.com/public/v1/documents/{document_id}/send
. Use thesilent: true
parameter so that you don't send any emails to recepients. - Create a document link:
POST https://api.pandadoc.com/public/v1/documents/{document_id}/session
. Use theid
from the response to create a session.
The optimal flow we recommend is as follows:
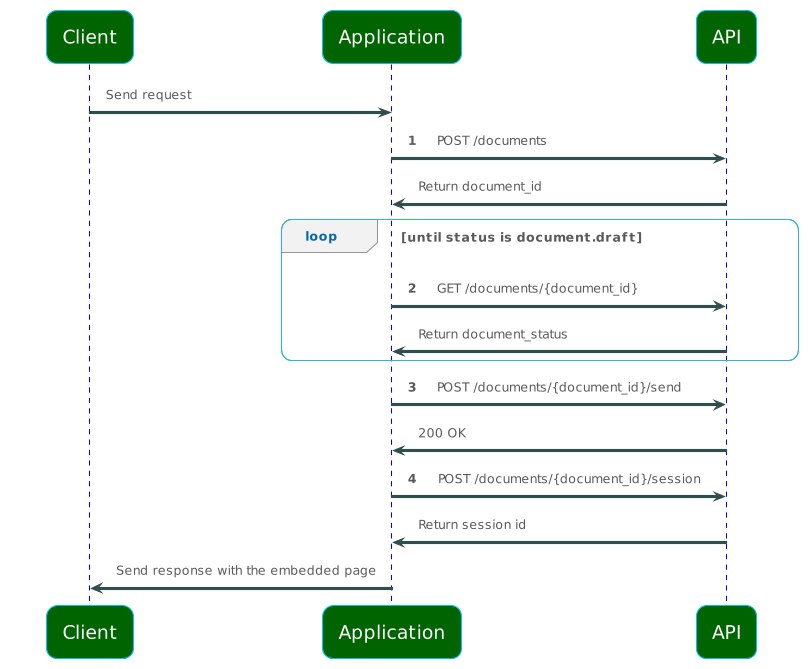
Embed document
Use the session_id
from the previous section within an HTML iframe like this:
<iframe src="https://app.pandadoc.com/s/{session_id}/"></iframe>
Identity verification
Any user who views a document within the generated session id can act on behalf of the recipient you created a view session for. It's your responsibility to verify the viewer identity.
Embedded document JavaScript events
When embedding a PandaDoc document, you may wish to perform custom application events based on viewer activity. For example, when the document is completed, redirect a user to another application page. That's where view session JavaScript events come in handy.
There are three available events:
session_view.document.loaded
- the document loaded.session_view.document.completed
- a document recipient filled in the assigned fields and finalized the document. At this point, the document can be in the Viewed status in case there are several Signers in the document or in the Completed status in case there is only one Signer or the last Signer has finalized the document.session_view.document.exception
- an error occurred while finalizing the document.
Here's a snippet of how you can register an event handler and process these events. Make sure to replace the iframe source URL with your own session ID:
<!doctype html>
<html>
<body>
<iframe src="https://app.pandadoc.com/s/{id}/" width="800" height="800"></iframe>
<script>
window.addEventListener('message', (event) => {
const type = event.data && event.data.type;
const payload = event.data && event.data.payload;
switch (type) {
case 'session_view.document.loaded':
console.log('Session view is loaded');
break;
case 'session_view.document.completed':
console.log('Document is completed');
console.log(payload);
break;
case 'session_view.document.exception':
console.log('Exception during document finalization');
console.log(payload);
}
});
</script>
</body>
</html>
Updated 29 days ago